반응형
https://www.acmicpc.net/problem/18221
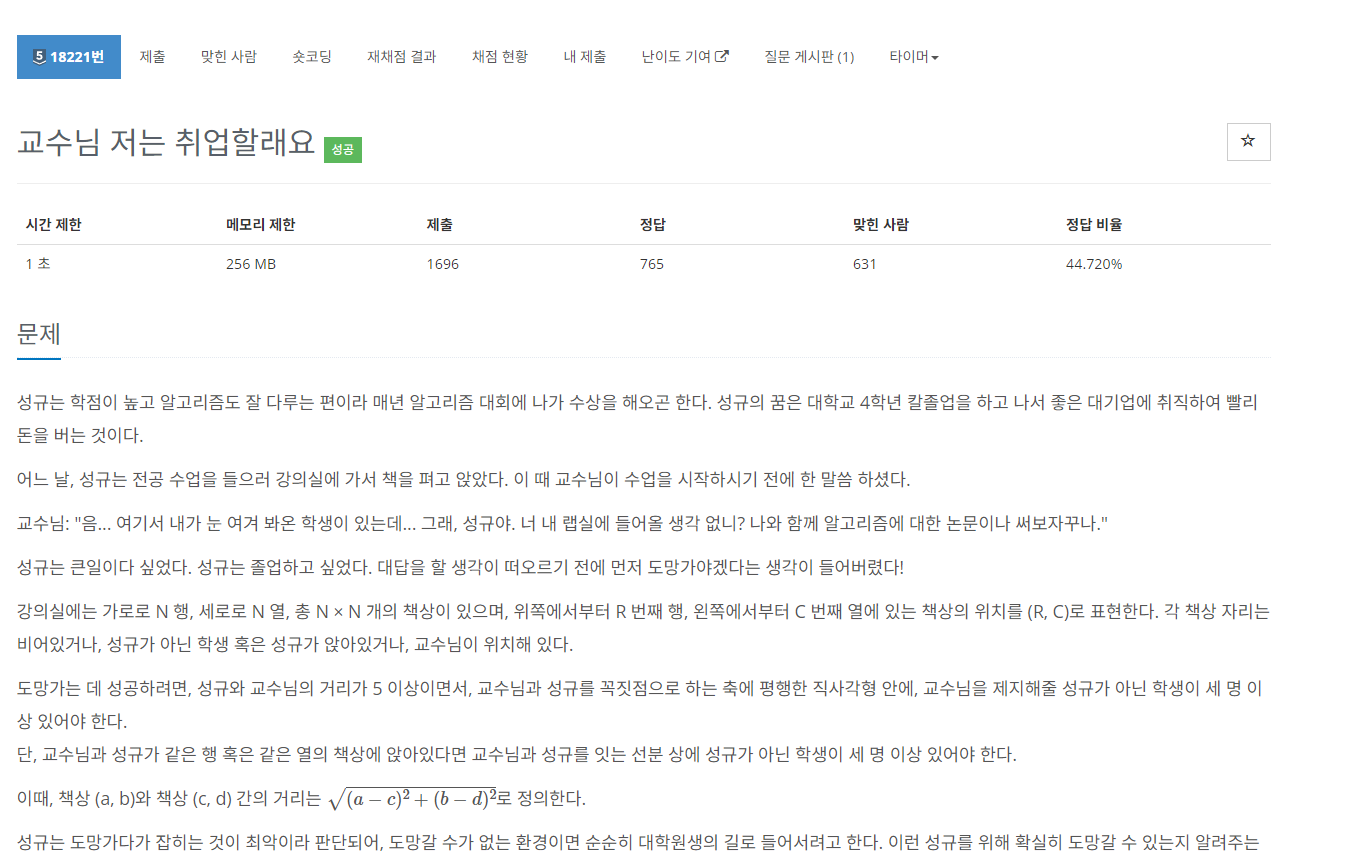
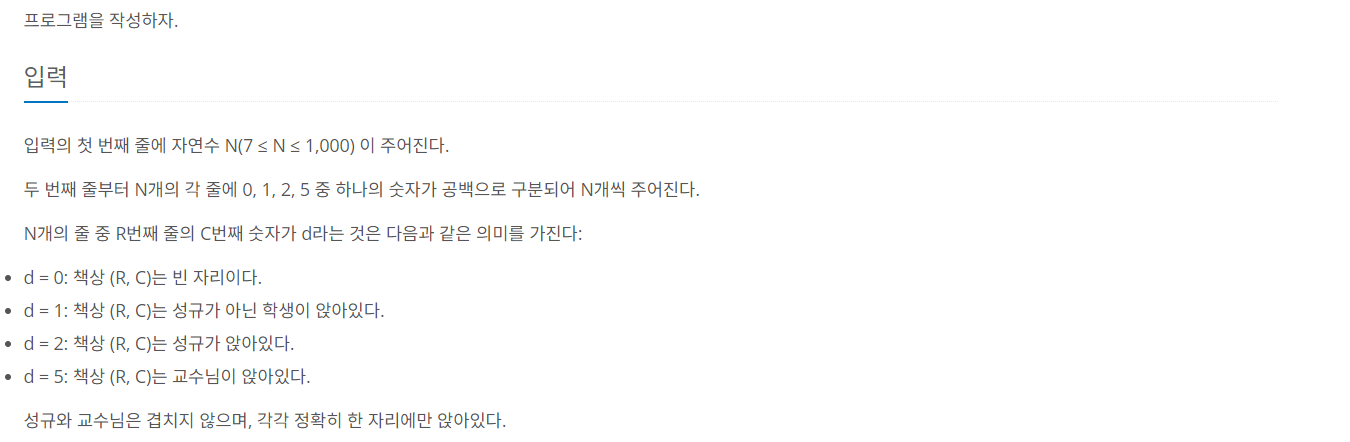
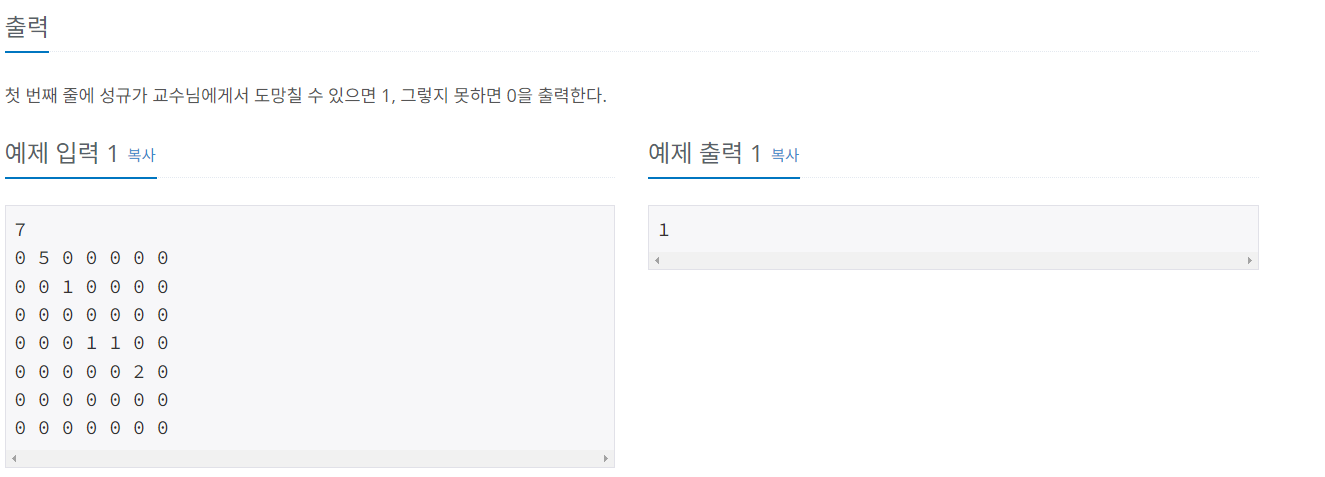
#문제 간단 정리
#문제 해결 방법
문제그대로 구현하면된다
#전체 코드
#include <iostream>
#include <vector>
#include <utility>
#include <climits>
#include <cmath>
using namespace std;
vector<vector<int>> board;
pair<int, int> prof, sung;
int n;
// 학생을 세는 함수
bool CountingStudents(int ys, int ye, int xs, int xe, bool sameLine) {
int count = 0;
if (sameLine) {
// 같은 행 또는 같은 열일 경우
if (xs == xe) { // 같은 행
for (int j = ys; j <= ye; j++) {
if (board[xs][j] == 1) {
count++;
}
}
} else { // 같은 열
for (int i = xs; i <= xe; i++) {
if (board[i][ys] == 1) {
count++;
}
}
}
} else {
// 직사각형 내 모든 셀
for (int i = xs; i <= xe; i++) { // 행 반복
for (int j = ys; j <= ye; j++) { // 열 반복
if (board[i][j] == 1) {
count++;
}
}
}
}
return count >= 3;
}
int main() {
cin >> n;
board.resize(n, vector<int>(n));
// 입력 받기 및 교수님과 성규의 위치 찾기
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cin >> board[i][j];
if (board[i][j] == 5) prof = {i, j};
if (board[i][j] == 2) sung = {i, j};
}
}
// 거리 계산 (제곱 거리 사용)
int dist = pow(abs(sung.first - prof.first), 2) + pow(abs(sung.second - prof.second), 2);
if (dist >= 25) {
// 교수님과 성규의 위치를 기준으로 직사각형 정의
int xs = min(prof.first, sung.first);
int xe = max(prof.first, sung.first);
int ys = min(prof.second, sung.second);
int ye = max(prof.second, sung.second);
// 같은 행 또는 같은 열인지 확인
bool sameLine = false;
if (prof.first == sung.first || prof.second == sung.second) {
sameLine = true;
}
// 학생 수 세기
if (CountingStudents(ys, ye, xs, xe, sameLine)) {
cout << 1 << '\n';
return 0;
} else {
cout << 0 << '\n';
return 0;
}
} else {
cout << 0 << '\n';
return 0;
}
return 0;
}
반응형
'[백준] > C++' 카테고리의 다른 글
백준 7861번 Longest Ordered Subsequence [C++] (0) | 2024.10.05 |
---|---|
백준 6207번 Cow Picnic [C++] (0) | 2024.10.04 |
백준 15812번 침략자 진아 [C++] (0) | 2024.10.02 |
백준 24595번 Rise and Fall [C++] (0) | 2024.10.01 |
백준 15423번 Canonical Coin System [C++] (1) | 2024.09.22 |
반응형
https://www.acmicpc.net/problem/18221
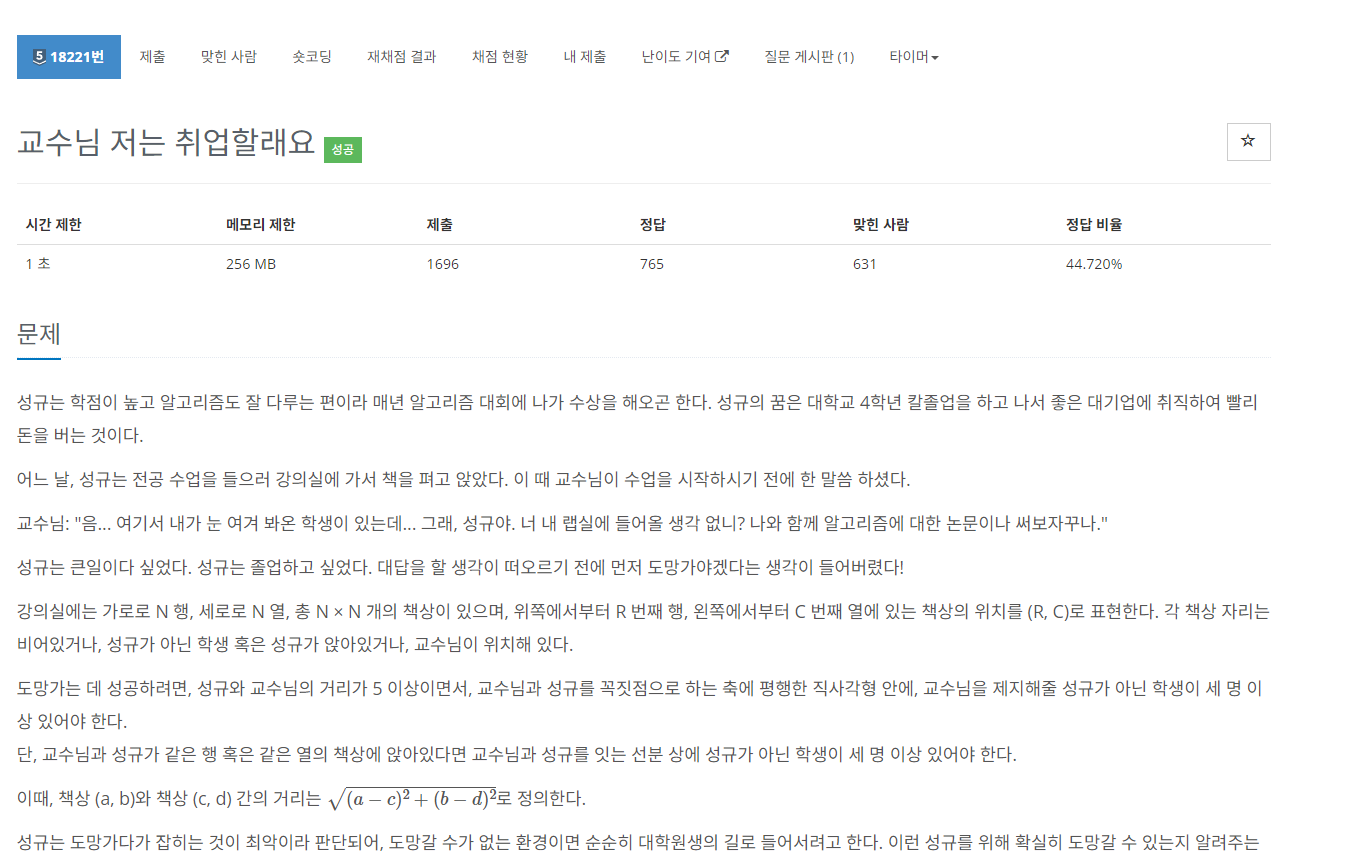
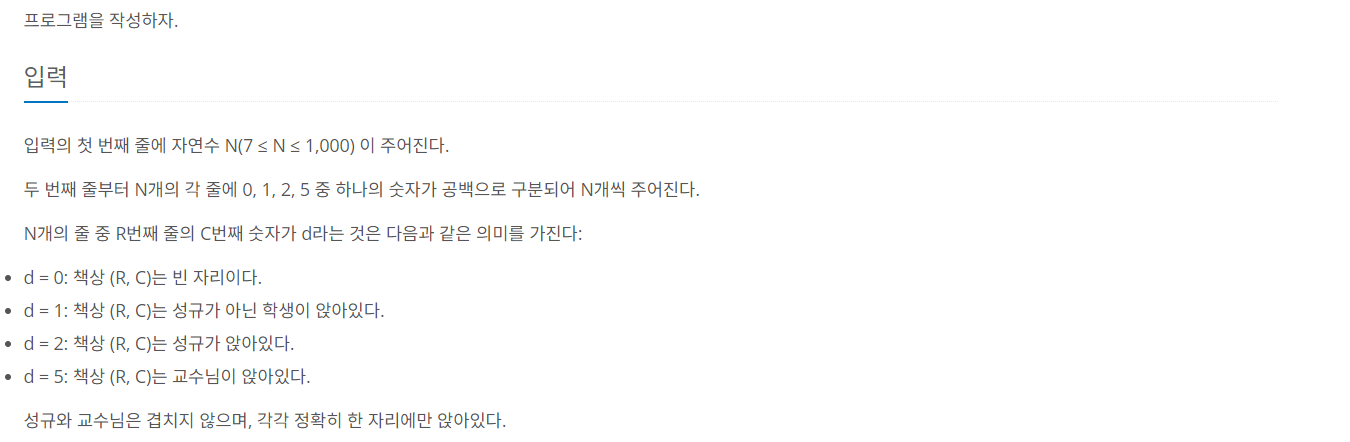
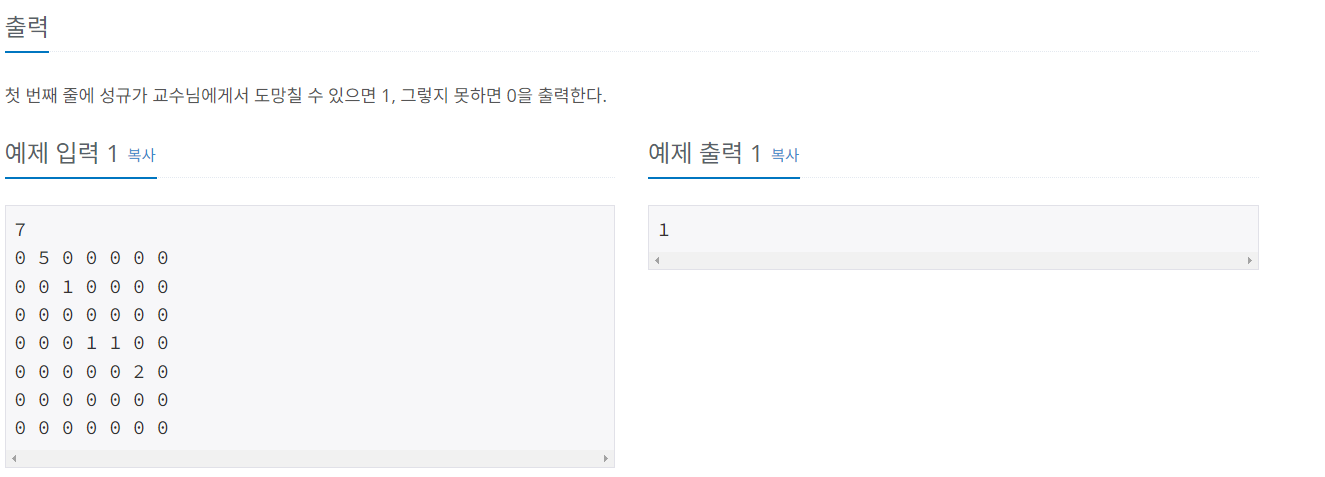
#문제 간단 정리
#문제 해결 방법
문제그대로 구현하면된다
#전체 코드
#include <iostream>
#include <vector>
#include <utility>
#include <climits>
#include <cmath>
using namespace std;
vector<vector<int>> board;
pair<int, int> prof, sung;
int n;
// 학생을 세는 함수
bool CountingStudents(int ys, int ye, int xs, int xe, bool sameLine) {
int count = 0;
if (sameLine) {
// 같은 행 또는 같은 열일 경우
if (xs == xe) { // 같은 행
for (int j = ys; j <= ye; j++) {
if (board[xs][j] == 1) {
count++;
}
}
} else { // 같은 열
for (int i = xs; i <= xe; i++) {
if (board[i][ys] == 1) {
count++;
}
}
}
} else {
// 직사각형 내 모든 셀
for (int i = xs; i <= xe; i++) { // 행 반복
for (int j = ys; j <= ye; j++) { // 열 반복
if (board[i][j] == 1) {
count++;
}
}
}
}
return count >= 3;
}
int main() {
cin >> n;
board.resize(n, vector<int>(n));
// 입력 받기 및 교수님과 성규의 위치 찾기
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cin >> board[i][j];
if (board[i][j] == 5) prof = {i, j};
if (board[i][j] == 2) sung = {i, j};
}
}
// 거리 계산 (제곱 거리 사용)
int dist = pow(abs(sung.first - prof.first), 2) + pow(abs(sung.second - prof.second), 2);
if (dist >= 25) {
// 교수님과 성규의 위치를 기준으로 직사각형 정의
int xs = min(prof.first, sung.first);
int xe = max(prof.first, sung.first);
int ys = min(prof.second, sung.second);
int ye = max(prof.second, sung.second);
// 같은 행 또는 같은 열인지 확인
bool sameLine = false;
if (prof.first == sung.first || prof.second == sung.second) {
sameLine = true;
}
// 학생 수 세기
if (CountingStudents(ys, ye, xs, xe, sameLine)) {
cout << 1 << '\n';
return 0;
} else {
cout << 0 << '\n';
return 0;
}
} else {
cout << 0 << '\n';
return 0;
}
return 0;
}
반응형
'[백준] > C++' 카테고리의 다른 글
백준 7861번 Longest Ordered Subsequence [C++] (0) | 2024.10.05 |
---|---|
백준 6207번 Cow Picnic [C++] (0) | 2024.10.04 |
백준 15812번 침략자 진아 [C++] (0) | 2024.10.02 |
백준 24595번 Rise and Fall [C++] (0) | 2024.10.01 |
백준 15423번 Canonical Coin System [C++] (1) | 2024.09.22 |